#77484
๐ Question ?
Description
Lotto 6/45(Hereinafter 'Lotto') is a popular lottery game where six numbers are drawn from a pool of 45 numbers. The lottery prize tiers are as follows1:
Prize TiersRequirement1 | All six numbers match |
2 | Five numbers match |
3 | Four numbers match |
4 | Three numbers match |
5 | Two numbers match |
6 (no prize) | All other cases |
You bought a lotto ticket and have been waiting for the draw. However, your little brother drew scribbles all over your ticket so that you can't make out some of its numbers. After the draw, you wanted to figure out the highest prize you could have won as well as the lowest prize you could have got.
Suppose that your ticket contained the following set of numbers: 44, 1, 0, 0, 31 25, where 0s represent the numbers on your ticket that you can't make out. If the six winning lottery numbers are 31, 10, 45, 1, 6, and 19, then the following shows the highest and the lowest prize you could have won.
Highest prize | 31 | 0โ10 | 44 | 1 | 0โ6 | 25 | four matching numbers, wins the 3rd prize |
Lowest prize | 31 | 0โ11 | 44 | 1 | 0โ7 | 25 | two matching numbers, wins the 5th prize |
- The order in which the numbers are drawn is irrelevant.
- Had the numbers you can't make out been 10 and 6, you could have won the 3rd prize.
- There are other possibilities where you could have won the 3rd prize. However, you would not have won a prize higher than the 3rd one.
- Had the numbers you can't make out been 11 and 7, you could have won the 5th prize.
- There are other possibilities where you could have won the 5th prize. However, you would not have won any prize (Tier 6).
Suppose parameters lottos and win_nums are given, where lottos is an array containing the numbers on your ticket and win_nums an array containing the winning numbers. Please write a function solution that returns the highest prize tier and the lowest prize tier that you could have won.
Constraints- lottos is an integer array whose length is 6.
- The elements of lottos are integers between 0 and 45.
- 0 represents the number you can't make out.
- Other than 0s, no integers in lottos overlap.
- The elements of lottos may not be arranged in a certain order.
- win_nums is an integer array whose length is 6.
- The elements of win_nums are integers between 1 and 45.
- No integers in win_nums will overlap.
- The elements of win_nums may not be arranged in a certain order.
https://school.programmers.co.kr/learn/courses/30/lessons/77484
๐งฉ Thought Process
- lottos์ ๋ชจ๋ ๊ฐ์ for๋ฌธ์ ๋๋ ค ํ์ธ ํ๋ค.
- 0์ ์๋ ๋จผํธ๋ก ๊ฐ์ง ๊ฒฝ์ฐ์๋ max_wins์ 1์ ๋ํด ์ฃผ์๋ค. ์ด ๊ฐ์ ์ถ๊ฐ์ ์ผ๋ก ๋ง์ถ์ ์๋ ๊ฐ์ ๋งํ๋ค.
- 0์ด ์๋ ๊ฒฝ์ฐ์๋ ๋ก๋๋น์ฒจ ์ซ์์ ๋ชจ๋ ๋น๊ต๋ฅผ ํด์ฃผ๊ณ ๊ฐ์ ์ซ์๊ฐ ์์ผ๋ฉด fixed_wins์ 1์ ๋ํด์ค๋ค.
- ๊ฐ์ ์ซ์๊ฐ ๋์ฌ ์ต์ ๊ฐ์๋ fixed_wins์ ์ ์ฅ๋ ์์ผ ๊ฒ์ด๊ณ ์ต๋ ๊ฐ์๋ fixed_wins+max_wins์ผ ๊ฒ์ด๋ค.
- ๋ฆฌํด ํด์ผํ๋ ๊ฐ์ ๋ง์ถ ๋ก๋ ๊ฐ์๊ฐ ์๋ ๋ง์ถ ๋ก๋ ๊ฐ์์ ๋ฐ๋ฅธ ๋น์ฒจ์ก ์์์ด๊ธฐ ๋๋ฌธ์ ์ด๋ฅผ ๋ณํํด์ฃผ๋ ํจ์๋ฅผ ๊ฐ๋จํ๊ฒ ๋ฐ๋ก ๋ง๋ค์ด์ฃผ ์๋ค.
๐ Answer
class Solution {
fun solution(lottos: IntArray, win_nums: IntArray): IntArray {
var fixed_wins:Int = 0
var max_wins:Int = 0
for (i in lottos.indices) {
if (lottos[i] == 0) {
max_wins += 1
} else {
for (j in win_nums.indices) {
if (lottos[i] == win_nums[j]) {
fixed_wins += 1
}
}
}
}
return intArrayOf(prizeTier(fixed_wins+max_wins), prizeTier(fixed_wins))
}
fun prizeTier(wins: Int):Int {
return when(wins) {
6 -> 1
5 -> 2
4 -> 3
3 -> 4
2 -> 5
else -> 6
}
}
}
๐ Result
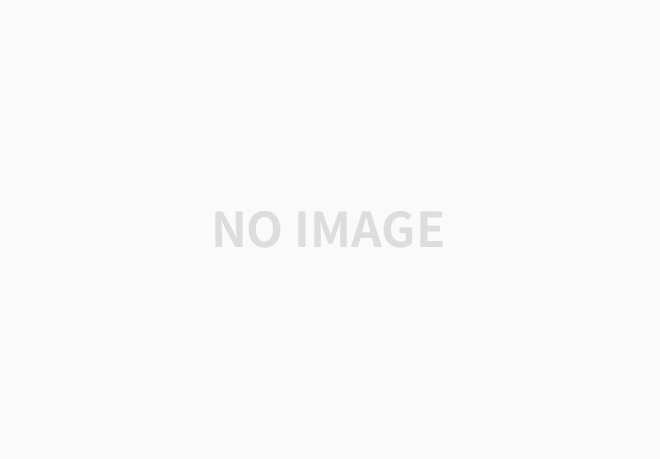
๐ Comment
indices๋ฅผ ์ฌ์ฉํ๋ฉด i๊ฐ ๊ทธ ์ธ๋ฑ์ค์ ํด๋นํ๋ ์๋ ๋จผํธ ๊ฐ์ ๊ฐ์ง ๊ฒ์ด๋ผ๊ณ ์ฐฉ๊ฐ์ ํด์ ์ค๊ฐ์ ์๊ฐ์ด ๊ฑธ๋ ธ๋ค.
array[i]๋ฅผ ํด์ฃผ์ด์ผํ๊ณ indices๋ฅผ ์ฌ์ฉํ๋ฉด i ๋ ์ธ๋ฑ์ค ๋ง์ ๋ฆฌํดํ ๋ค๋ ๊ฒ์ ๊ธฐ์ตํด์ฃผ์ด์ผ๊ฒ ๋ค.
'Algorithm > Kotlin' ์นดํ ๊ณ ๋ฆฌ์ ๋ค๋ฅธ ๊ธ
[ํ๋ก๊ทธ๋๋จธ์ค โข ์ฝํ๋ฆฐ] ๊ธฐ์ฌ๋จ์์ ๋ฌด๊ธฐ #136798 (0) | 2024.01.15 |
---|---|
[ํ๋ก๊ทธ๋๋จธ์ค โข ์ฝํ๋ฆฐ] ๋ง์น ํ๊ธฐ #161989 (2) | 2024.01.05 |
[ํ๋ก๊ทธ๋๋จธ์ค โข ์ฝํ๋ฆฐ] Generating Prime Number #12977 (0) | 2023.12.29 |
[ํ๋ก๊ทธ๋๋จธ์ค โข ์ฝํ๋ฆฐ] ๋ชจ์๊ณ ์ฌ ์์ ํ์ #42840 (0) | 2023.12.27 |
[ํ๋ก๊ทธ๋๋จธ์ค โข ์ฝํ๋ฆฐ] 2016๋ #12901 (0) | 2023.12.19 |